Creating Dynamic and Interactive Pages with Velo by Wix
Velo by Wix is a powerful development platform that allows you to create dynamic, interactive, and highly customized web pages using JavaScript, APIs, and more. This blog will guide you through advanced techniques for using Velo by Wix to create dynamic and interactive pages, ensuring a rich and engaging user experience.
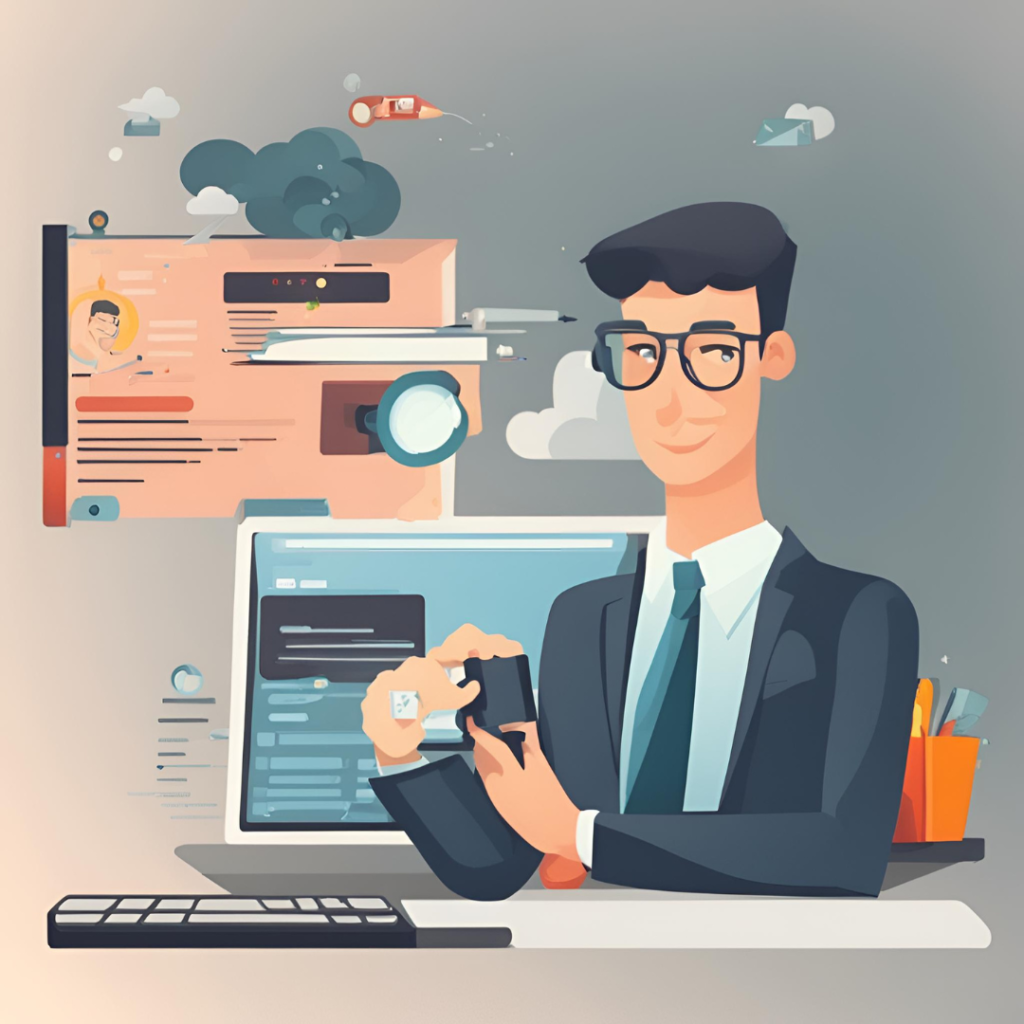
1. Getting Started with Velo by Wix
Before diving into advanced techniques, ensure you have a basic understanding of Velo by Wix and have enabled Developer Mode on your Wix site.
Enabling Developer Mode:
- Open your Wix site in the editor.
- Click on “Dev Mode” at the top of the editor.
- Toggle the switch to enable Developer Mode.
This action will open the Code Panel, where you can write JavaScript code, create collections, and manage your site’s backend.
2. Using Data Collections and Dynamic Pages
Data collections are the backbone of dynamic content in Wix. They allow you to store structured data and display it dynamically on your site.
Creating Data Collections:
- In the Wix Editor, click on the “Content Manager.”
- Select “Add New Collection” and define the fields you need.
- Populate the collection with your data.
Setting Up Dynamic Pages:
- Go to the Pages menu, select “Dynamic Pages,” and create a new dynamic page.
- Link the dynamic page to your data collection.
- Design the page layout. The dynamic content will automatically populate based on the data in your collection.
Example: Creating a Dynamic Blog Post Page
- Create a collection named “BlogPosts” with fields like Title, Content, Author, and Date.
- Add a dynamic page linked to the “BlogPosts” collection.
- Design the dynamic page template to display the blog post details.
3. Enhancing Interactivity with JavaScript
JavaScript is a core component of Velo by Wix, allowing you to add interactivity to your web pages.
Adding Event Handlers:
- Select an element on your page (e.g., a button).
- Open the Code Panel and write an event handler for the element.javascript
$w.onReady(function() { $w('#myButton').onClick(() => { console.log("Button clicked!"); // Add your custom logic here }); });
Example: Creating a Filterable Gallery:
- Create a collection named “GalleryItems” with fields like Image, Title, and Category.
- Add a gallery to your page and link it to the “GalleryItems” collection.
- Add buttons for each category and use JavaScript to filter the gallery items based on the selected category.javascript
$w.onReady(function() { $w('#categoryButton').onClick(() => { let category = "Nature"; // Example category $w('#gallery').setFilter(wixData.filter().eq('category', category)); }); });
4. Connecting to External APIs
Velo by Wix allows you to connect your site to external APIs, enabling you to fetch and display data from other sources.
Example: Displaying Weather Data:
- Sign up for a weather API service (e.g., OpenWeatherMap) and get your API key.
- Write backend code to fetch weather data from the API.javascript
// backend/weather.jsw import { fetch } from 'wix-fetch'; export function getWeather(location) { const apiKey = 'YOUR_API_KEY'; const url = `https://api.openweathermap.org/data/2.5/weather?q=${location}&appid=${apiKey}`; return fetch(url) .then(response => response.json()) .then(data => { return { temperature: data.main.temp, description: data.weather[0].description }; }); }
- Call the backend function from your page code and display the weather data.javascript
import { getWeather } from 'backend/weather.jsw'; $w.onReady(function() { getWeather('New York').then(weather => { $w('#temperature').text = `${weather.temperature}°C`; $w('#description').text = weather.description; }); });
5. Implementing Real-Time Updates
Real-time updates can significantly enhance the user experience by providing live data updates without refreshing the page.
Example: Live Chat Feature:
- Add a chat collection to store messages.
- Use real-time API services like Firebase to listen for new messages and update the chat interface dynamically.javascript
import { realTime } from 'wix-realtime'; $w.onReady(function() { realTime.onMessage('chat', (message) => { $w('#chatBox').appendText(`${message.sender}: ${message.text}\n`); }); }); // Send a message $w('#sendButton').onClick(() => { let message = { sender: $w('#username').value, text: $w('#messageInput').value }; realTime.sendMessage('chat', message); });
6. Optimizing Performance and User Experience
Performance is crucial, especially for dynamic and interactive sites. Here are some tips to ensure your site runs smoothly:
Lazy Loading:
- Load images and other media assets only when they enter the viewport.javascript
$w.onReady(function() { $w('#image').src = 'path/to/image.jpg'; // Set the source when the image is about to enter the viewport });
Debouncing User Input:
- Use debouncing techniques to limit the number of API calls or heavy computations in response to user input.javascript
function debounce(func, wait) { let timeout; return function(...args) { clearTimeout(timeout); timeout = setTimeout(() => func.apply(this, args), wait); }; } $w('#searchInput').onInput(debounce((event) => { // Perform search search(event.target.value); }, 300));
Optimizing Data Queries:
- Fetch only the necessary data and use pagination for large datasets.javascript
import wixData from 'wix-data'; $w.onReady(function() { wixData.query('BlogPosts') .limit(10) // Fetch only 10 items at a time .find() .then(results => { // Display results }); });
Conclusion:
Creating dynamic and interactive pages with Velo by Wix involves utilizing data collections, JavaScript, external APIs, real-time updates, and performance optimization techniques. By following these best practices, you can enhance your site’s functionality and user experience, making it both engaging and efficient.
If you have any questions or need further assistance, feel free to reach out. Happy coding!